I am learning about STM32 programming and trying to implement simple asynchronous serial communication using USART peripheral on GPIO pins. The amazing book is not on fire ebook reader.
The HAL manual describes how to use HAL USART drivers:
- Declare a USART_HandleTypeDef structure
- Implement HAL_USART_MspInit()
- Enable USART & GPIO clocks
- Configure GPIOs
- Program the communication parameters in the USART_InitTypeDef
- Call HAL_USART_Init()
As I wrote my code, I declared the USART_HandleTypeDef, instinctively filled my USART_InitTypeDef structure and started to fill the HandleTypeDef:

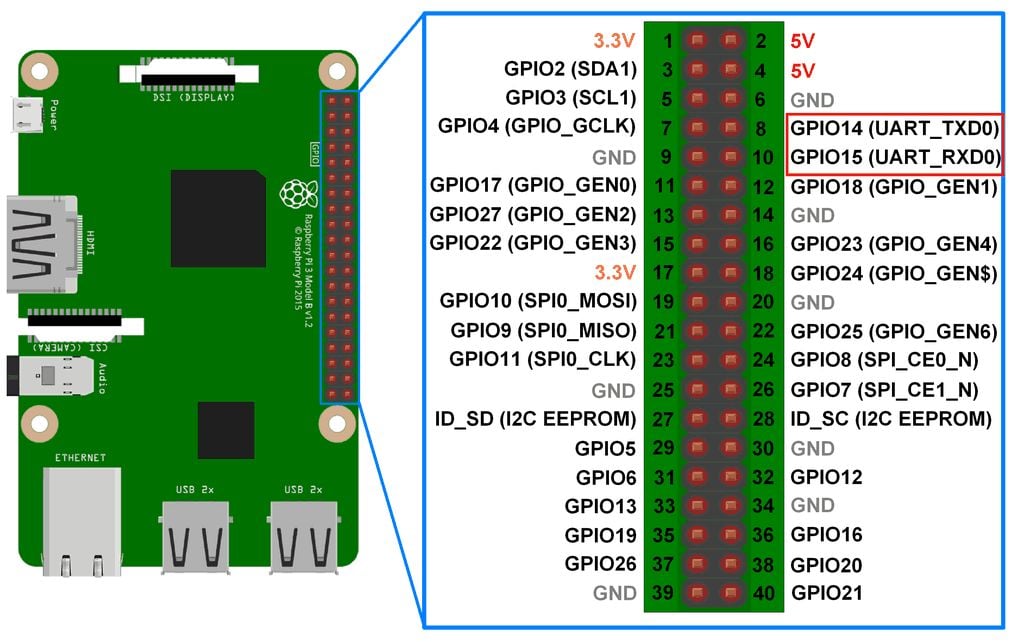
Using The UART In Your C Code (This is based on the example code given here ). Update from Maurice Garcia in the comments – If you are using PI model 3 do not use /dev/ttyAMA0 this is assigned to bluetooth, use /dev/ttyS0.
I then noticed that there's many data fields to fill. Referring to code examples in the manual and on the web, I noticed nobody actually defines all of the USART_HandleTypeDef fields - they somehow combine the HandleTypeDef and InitTypeDef in one step, like this:
How does this work? What part of C syntax do I have to learn, to understand where did that UartHandle.Init.xxx come from?Is it possible to do it 'the long way', as I planned to? If I don't fill every datafield of HandleTypeDef, where do they get initialized?
PS. I am not using STM32 recommended IDEs or CubeMX, working on Linux, using PlatformIO. Board: STM32F746 discovery kit
PPS. I am really unsure whether to put this question here or on electronics stack. Please correct me or move the question there if it's not suitable for this stackexchange.
1 Answer
How does this work? What part of C syntax do I have to learn, to understand where did that UartHandle.Init.xxx come from?
This is basic C struct syntax. The USART_HandleTypeDef
struct contains an instance of a USART_InitTypeDef
struct named Init
. You can think of it as a nested struct. You can reference the members of nested structs with repeated '.
's. Note that, the Init
member is NOT a pointer to a USART_InitTypeDef
struct. It's literally a complete USART_InitTypeDef
instance contained within a USART_HandleTypeDef
instance.
Is it possible to do it 'the long way', as I planned to?
Yes, except your code contains an error. Bin weevils sign in. You need to make the assignment like this.
Remember that the Init
member of USART_HandleTypeDef
is not a pointer but a complete struct. Therefore you need to assign it with a complete struct, not a pointer.
But realize that when you define your UsartInit
variable, you are allocating space for an instance of the struct. If UsartInit
is a function local variable then that space is likely on the stack. Your initialization statements are initializing your copy of the struct. Then when you assign UsartInit
to UsartHandle.Init
the compiler creates code that will copy the entire contents of the struct. After the copy, if your UsartInit
is a local variable, it will go out of scope and be deallocated.
Uart Driver C Code Syntax Highlighting
It's really not necessary to define and allocate space for your own USART_InitTypeDef
struct and then copy the entire struct into UsartHandle.Init
. UsartHandle
already contains space allocated for its USART_InitTypeDef
member. So it's more efficient to simply initialize the UsartHandle.Init
member directly, like the ST example code does.
If I don't fill every datafield of HandleTypeDef, where do they get initialized?
You don't need to fill every datafield of USART_HandleTypeDef
. Refer to the HAL Reference Manual to learn what you need to initialize. You probably need to initialize only the Instance
and Init
members. The remaining members are used internally by the HAL USART driver and they will be initialized and used by the driver functions (you can think of them as private variables if that helps). The designer of the API named that struct member 'Init' as a cue to you that this is what you need to initialize. The ST example code provides further evidence of what you need to initialize. Foscam ip camera tool for mac download.
[Several experienced developers on Stack Overflow advise against using the ST HAL and encourage people to develop their own drivers based on the device's Reference Manual. Realize that these developers have years of experience, they've worked with a variety of microcontroller families and peripherals, and they're capable of understanding the Reference Manual and writing drivers from scratch. I agree that the ST HAL adds some bloat that might be detrimental to some applications. But I disagree that beginners should avoid using the ST HAL. The ST HAL works well enough for many applications and it is easier for beginners to use than writing their own drivers from scratch (especially given the many examples provided with the HAL).]
kkrambokkramboNot the answer you're looking for? Browse other questions tagged csyntaxembeddedstm32hal or ask your own question.
This is a simple MAVLink to UART interface example for *nix systems that can allow communication between Pixhawk and an offboard computer.
His research in biostatistics is on computer intensive inference and surveillance methods that involve screening methodologies, with their associated laboratory tests, and in obtaining more accurate testing results that use existing technologies. Principles of biostatistics solutions pdf. Marcello Pagano is Professor of Statistical Computing in the Department of Biostatistics at the Harvard School of Public Health.
This example will receive one MAVLink message and send one MAVLink message.
Connect the USB programming cable to your Pixhawk.
If you want to be able to interact with this example in Pixhawk's NuttX shell, you'll need a Telemetry Radio or an FTDI developer's cable. See the Exploration section below for more detail.
A free version of DeskFX Audio Enhancing software is available for non-commercial use. https://queenclever285.weebly.com/graphic-equalizer-for-mac-download.html.
Also Note: Using a UART (serial) connection should be preferred over using the USB port for flying systems. The reason being that the driver for the USB port is much more complicated, so the UART is a much more trusted port for flight-critical functions. To learn how this works though the USB port will be fine and instructive.
Audacity per mac download free. With the latest version of Audacity, you need not get the VST Enabler. Acon Digitals – best for livening the vocal soundConclusionIt is simple to add effects to produce good sound. You just have to be resourceful and creative on using these free plugins.
Uart Driver C Code Syntax
You have to pick a port name, try searching for it with
Alternatively, plug in Pixhawk USB cable again and issue the command:
The device described at the bottom of dmesg's output will be the port on which the Pixhawk is mounted.
The Pixhawk USB port will show up on a ttyACM*
, an FTDI cable will show up on a ttyUSB*
.
Ships games free download. Ships 2017 Free Download PC Game setup in single direct link for Windows. It is an awesome simulation game. OceanOfGames, Ocean Of Games, OceanGames PC. Ships 2017 PC game 2016 Overview. Ships 2017 has been developed by Fragout and is published under the banner of PlayWay S.A. This game was released on 20 th October, 2016.
Run the example executable on the host shell:
To stop the program, use the key sequence Ctrl-C
.
Here's an example output:
There are a few things to explore past this example.
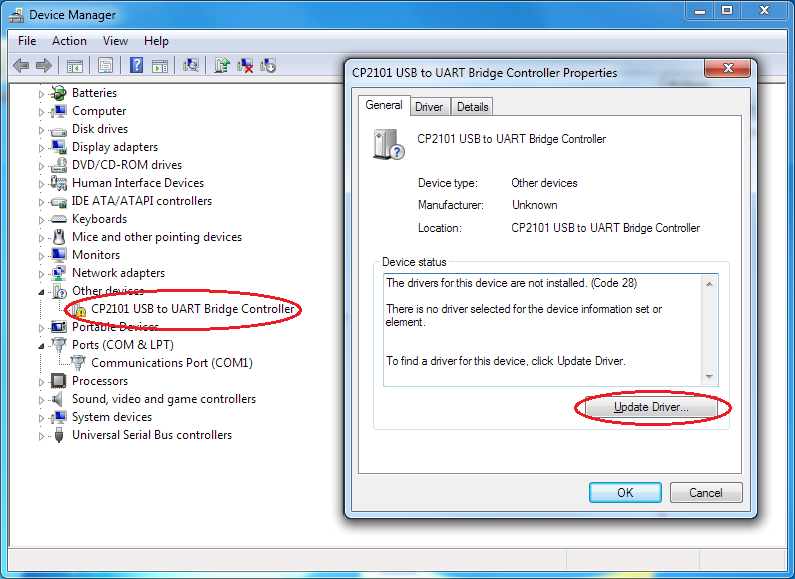
First you can connect via:
- a Telemetry Radio on TELEM1 or 2
- an FTDI cable on TELEM2 or Serial 4
Note
- Serial 5 can't be used to receive data without reconfiguration (its receive pin is occupied by a second NuttX shell).
- TELEM1 is typically dedicated to Telemetry Radio, but if you're using another port you will need to make sure it is not configured for use by another peripheral.
- If using FTDI with a TELEM port, connect all the pins to corresponding pins on port.
- If using FTDI with SERIAL4 connect the TX, RX GND and 5V pins (CTS, RTS need not be connected).
With this you'll be able to start a second port for communication, and leave the USB port available for viewing prints in the NuttX shell.
For steps 2 and 3 from the above tutorial, you'll use a different port. On the off-board computer side, the port might now be /dev/ttyUSB0
. On the Pixhawk side, here the port mappings are in the table below.
PX4 UART | NuttX UART |
---|---|
Telem 1 | /dev/ttyS1 |
Telem 2 | /dev/ttyS2 |
Serial 4 | /dev/ttyS6 |
Now add a print statement in the Pixhawk Firmware to see received messages. Build and upload this to Pixhawk.
Open the system terminal as described here: https://dev.px4.io/en/debug/system_console.html
On the off-board side, in another terminal run the c_uart_interface_example
executable. You should see output in the NuttX shell similar to this: Mac remote desktop free.
Past this, you can:
- Modify the received message data type
- Modify the sent message data type